By virtue of being a distributed ledger in a P2P network, the blockchain is relatively more secure than data that is on a central server. It is made more secure by the use of powerful cryptography and algorithms. So decentralised applications based on blockchain technology provide excellent security for various types of data.
Blockchain technology is being widely used in the domain of cryptocurrency. A number of digital cryptocurrencies have gained prominence and are being shared throughout the world despite much criticism and controversies. These include Bitcoin, Ethereum, LiteCoin, PeerCoin, GridCoin, PrimeCoin, Ripple, Nxt, DogeCoin, etc.
These blockchain based cryptocurrencies do not have any intermediate bank or payment gateway to record the log of the transactions. That is the main reason why many countries are not recognising cryptocurrencies as valid money transactions. In spite of this, these blockchain based cryptocurrencies are rather popular and are used because of their many security features.
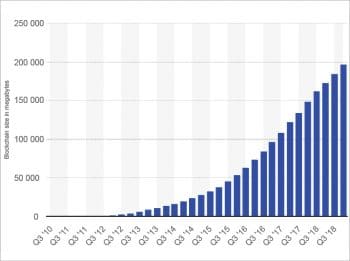
The blockchain network has a block of records, in which every record is associated with dynamic cryptography so that all the transactions can be encrypted without any probability of sniffing or hacking attempts.Case studies
In the current scenario, blockchain technology is more focused towards cryptocurrencies in which a distributed ledger is maintained for the transactions. The distributed ledger refers to a replicated and synchronised digital asset shared by users at multiple locations and on various devices so that third party manipulation isn’t possible. For example, if a bank uses the distributed ledger with blockchain technology, it can enforce a higher degree of security. If that bank has one million customers then the records of their transactions will be stored on those one million devices. In fact, a hacker will have to hack one million devices in real-time rather than a single server. This is the major advantage of using the decentralised blockchain technology.
In case of a centralised application, if a hacker penetrates the server of a bank, then the details and records of all the customers can be copied. That is the main reason why government agencies should focus on decentralising their Web based applications.
Using Blockchain technology, government servers used for land registry, citizen information (including Aadhaar), Permanent Account Number (PAN) and many others can be secured using decentralised apps.
The blockchain based decentralised applications can be used for the following:
- Birth, marriage and death certificates
- Asset and land registry
- Digital identity of government documents
- Incorporation services
- Notarised documents
- Personalised government services
- Social welfare and benefits
- Taxation
- Polling, voting at assembly elections, etc
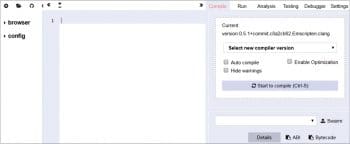
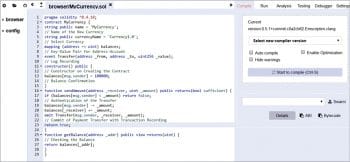
Decentralised application (dApp) with the secured blockchain
The decentralised application (dApp) executes on the distributed channels so that hacking the app is next to impossible. A traditional centralised application is deployed on a single server. The main limitation with this approach is that if the central server is hacked then everything can be damaged or copied from it. In case of decentralised applications, there is no single server but rather storage is done on all the client devices so that the replication of the transaction can be done with maximum availability of transaction records. In the case of a decentralised application, the hacker will have to crack all the devices associated with that application and that will be very difficult in real-time using smart contracts. In a smart contracts based dApp, dynamic token sharing is implemented so that the transactions will have the maximum security measures.
Smart contracts
Smart contract programming is required for global transactions. This means that transactions can be executed between people who can’t communicate because they are on different continents, speak different languages and practice different traditions. Smart contracts automatically validate transactions and business dealings between the people who can’t understand each other’s languages.
Free and open source tools for blockchain development
Listed below are some of the leading FOSS tools for blockchain development.
Hydrachain
https://github.com/HydraChain/hydrachain
- Users can create permissioned distributed ledgers
- They can set up a private chain
- Fully compatible with the Ethereum protocol
Multichain
https://www.multichain.com/
- Compatible with Bitcoin
- Fully customisable
- Fine-grained managed permissions
- Enables the rapid creation and deployment of a new blockchain
- Powerful data sharing and encryption
OpenChain
https://www.openchain.org/
- Digitally signed transactions
- Custom rule definitions for ledgers
- Robustness and fine validation
- Client server architecture
- Module design with real-time validations
- Immutability with security
Ethereum
https://www.ethereum.org/
- Smart wallets and smart money
- Supports the creation and development of users’ own cryptocurrency
- Security against third party intervention or downtime
- Execution of smart contracts
- Virtual shares with crowd fund and crowd sale
Corda
https://www.corda.net/
- Platform for blockchain and the distributed ledger
- Smart contracts
- Supports the development of distributed apps
- Notary infrastructure for sequencing and validation of transactions
- Flow based framework for negotiation and communication between the participants
Credits
https://credits.com/
- Smart contract programming
- Real-time monitoring of network transactions
- Web wallet with security using private and public keys
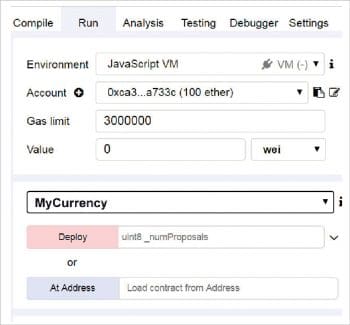
BigChainDB
https://www.bigchaindb.com/
- Big Data enabled blockchain database
- Decentralised management and control
- Dynamic management of digital assets
- Byzantine Fault Tolerance (BFT) for high performance computing applications
- Rich permissioning at each transaction
- Integration with MongoDB NoSQL for fast transaction processing with unstructured data
- Resistant to tampering; hence secure
Quorum
https://www.jpmorgan.com/global/Quorum
- Enterprise level smart contract and distributed ledger platform
- Peer permissioning
- High performance using Raft based consensus
- Exchange of private messages with secured contracts
- Fully customisable for large scale business and corporate applications
- Integration with CakeShop software development kit (SDK) for GUI enabled smart contracts, Quorum networks and APIs
There are other open source tools such as BigChainDB, Credits, Symbiont Assembly, Embark, Solidity, Truffle, etc, which interested readers can Google to learn more about.
Programming blockchain using open source development environments
Solidity
Solidity is a powerful, high-performance programming language for writing smart contracts. It follows the object-oriented programming paradigm with a higher degree of security and performance, which can be integrated with assorted blockchain platforms. The Solidity code is compiled and transformed to bytecode, which is executed on the Ethereum Virtual Machine (EVM). Solidity programming has the key base of many programming languages and scripts including Python, JavaScript and C++ so that it can be integrated with multiple environments and platforms for integration with blockchains. To work with Solidity, there are many integrated development environments (IDEs) and editors that can be used including Remix, EthFiddle and JetBrains.
To start with Solidity, use Remix, one of the powerful IDEs that is open source and also provides the Web based interface. Using the Web based interface of the Remix IDE, it is easy for developers to create smart contracts with blockchain programming.
The URL of the Web based remix IDE is remix.ethereum.org and it can be accessed directly from Web browsers for writing, compiling and executing the smart contracts.
In the following source code, a new currency MyCurrency is programmed using Solidity. The new currency with its own set of rules, protocols and security mechanism can be initialised during code customisation.
pragma solidity ^0.4.18; contract MyCurrency { string public name = ‘MyCurrency’; // Name of the New Currency string public currencyName = ‘Currency1.0’; // Select Currency mapping (address => uint) balances; // Key-Value Pair for Address-Account event Transfer(address _sender, address _receiver, uint256 _value); // Log Recording constructor() public { // Constructor on Creating the Contract balances[msg.sender] = 100000; // Balance Confirmation } function sendAmount(address _receiver, uint _amount) public returns(bool sufficient) { if (balances[msg.sender] < _amount) return false; // Authentication of the Transfer balances[msg.sender] -= _amount; balances[_receiver] += _amount; emit Transfer(msg.sender, _receiver, _amount); // Commit of Payment Transfer with Transaction Recording return true; } function getBalance(address _addr) public view returns(uint) { // Checking the Balance return balances[_addr]; } }
On clicking on the Deploy option in the Remix IDE, the code is executed and detailed logs of the transaction can be viewed.
The detailed logs of the blockchain transaction can be analysed. There are multiple parameters in the transaction log including the gas limit, which in smart contract programming refers to the amount of work or throughput associated with the transaction.
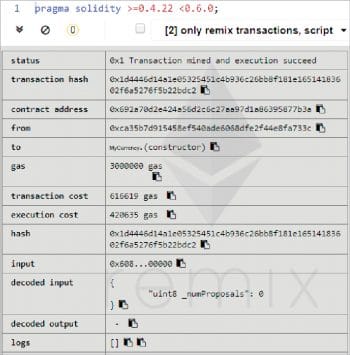
NodeJS and Web3JS
NodeJS is a cross-platform open source platform for JavaScript based programming. It can be used for multiple applications including blockchain development, smartphone applications, distributed Web applications, NoSQL processing, Big Data analytics, machine learning, Internet of Things (IoT) and many other topics related to advanced computing.
For blockchain programming, NodeJS is integrated with Web3JS, which is the set of libraries and tools for interaction with blockchain based connections.
The Web3JS platform for blockchains and dApp can be integrated with the following instructions:
npm: npm install web3 pure js: link the dist/web3.min.js meteor: meteor add ethereum:web3
After installation, the code in JavaScript and Server Side Scripts is written with the smart contracts and deployed for the secured applications.
Truffle
Truffle is another programming suite for the development of blockchain based smart contracts that can be installed with NodeJS and be executed on Ethereum Virtual Machine (EVM).
With the execution of the following instruction, the Truffle suite is associated with NPM:
npm install -g truffle
After the installation of Truffle, the installed version can be checked in the terminal as follows:
truffle version
A new project, MyBlockchain in Truffle can be mapped as follows:
mkdir MyBlockchain cd MyBlockchain truffle MyBlockchain
In Truffle, the directory structure given below is followed to code the application:
- contracts/: Source code for the smart contracts
- migrations/: Migration system and handlers for the smart contracts
- test/: Tests and JavaScript code
- truffle.js: Configuration file for Truffle
- MyBlockchain: Additional folders and files required for coding the blockchain
A new file <filename.sol> is created in the contracts/ directory for the base coding of smart contracts in the following format:
pragma solidity ^0.5.0; contract Mysmartcontract { } Sending Values function adopt(uint MyVar) public returns (uint) { require(MyVar >= 0 && MyVar <= 15); adopters[MyVar] = msg.sender; return MyVar; }
The compilation of Truffle code is done as follows:
truffle compile
Embark
The Embark framework provides the tools and libraries for the development of decentralised apps so that blockchain based implementation can be executed. Embark can be used as an alternate to Truffle. To work with Embark, you need to integrate Node Version Manager (NVM) with multiple versions of NodeJS.
NVM can be installed as follows:
curl -o- https://raw.githubusercontent.com/creationix/nvm/v0.33.11/install.sh | bash nvm install --lts nvm use --lts
Once NVM is installed, the toolkit of Embark can be associated as follows:
npm -g install embark
After installation, the initialisation instruction is executed for the new project, as follows:
embark new mysmartcontract cd mysmartcontract
By using ‘embark run’, the dashboard of Embark is invoked.
The token generation can be further written in code as follows:
pragma solidity ^0.4.25; import “openzeppelin-solidity/contracts/token/ERC20/ERC20.sol”; contract CryptoToken is ERC20 { string public name = “CryptoToken”; string public symbol = “MC”; uint256 public decimals = 18; constructor() public { } }
As in the above example, MC refers to the new currency symbol, which can be anything, depending on the requirements of the smart contract associated with the blockchain.
Scope for research and development
As blockchain based development is an emerging domain of research, there is a need to address different issues related to privacy and resource optimisation. In blockchain and other decentralised applications, the data is replicated to numerous devices and the issues of security and integrity do not arise. With the development and deployment of advanced algorithms, the performance of blockchain based implementations can be elevated further.