Flutter is a mobile UI framework from Google that helps to build native Android and iOS applications with a single codebase. This article discusses its numerous advantages.
Flutter is free and open source, and its creators state that it provides high-quality native interfaces on Android and iOS in record time. Flutter has been developed to enable the best mobile development experience on different platforms.
The biggest selling point of Flutter is the single codebase. Apps for both Android and iOS can be developed by writing the code just once. No prior experience in mobile development is required to get started with Flutter. As a result, you can start coding right away.
Flutter has been created to help developers to improve the application’s quality and speed up the pace of app development in order to reach more users. It is supported by various IDEs like Android Studio, VS Code and IntelliJ. Information on Flutter, such as the installation steps, features and the getting started guide can be easily found on the website https://flutter.io/.
Why Flutter?
Google I/O 2018 has officially declared Flutter to be ‘production ready’ with the framework being in the Beta 3 stage. The following features of Flutter make it a perfect choice for mobile app development.
- Faster development: Flutter provides a tool called ‘Hot Reload’ to help build features quickly, design UIs faster and enable swift bug fixes. An app can be built in record time with this super helpful tool.
- Native performance: Flutter incorporates the differences between the Android and iOS platforms such as the icons, fonts, navigation, etc, to make sure users get to enjoy a native experience on both platforms.
- Flexible UI: The UI experience of native platforms is not lost in Flutter and as a result, developers can provide features that focus on a native end user experience.
- Modern, reactive framework: Flutter comprises powerful and flexible APIs for gestures, animations, effects and a lot more. It has a rich set of platforms, layouts and foundation widgets.
- Highly customisable: Without the limitation of OEM widget sets, highly customisable and brand driven UIs can be developed.
The language for development
Dart is the language used for the development of Flutter apps. Even though it has been in use for a long time to create high-quality, critical apps for Android, iOS and the Web, Dart has gained popularity with the introduction of the Flutter framework. Dart is basically a client-optimised language with rich and powerful frameworks along with flexible tooling. It is well suited to reactive programming and supports asynchronous programming through language features and powerful APIs.
If you have never used Dart, fret not! It is a simple language that can be understood quickly and comes with solid documentation. You can start coding in Dart in no time. A new version of it — Dart 2, has been introduced recently with many new features. More about Dart can be found at the website https://www.dartlang.org/.
Flutter architecture
Flutter is based on the C++ engine that comprises Skia. The framework is based on this engine andcomprises widgets, animation, rendering, etc (source: https://flutter.io/technical-overview/).
Setting up the editor
To create your first Flutter app you’ll need to set up your editor. For this we need to install the following.
- Flutter SDK: This includes Flutter’s engine, widgets, tools and the Dart SDK.
- IDE: Flutter supports various IDEs like Android Studio, IntelliJ, VS Code, etc. You can work from the command line too.
- Plugins: Flutter and Dart plugins must be installed for your particular IDE.
See Flutter Installation and Setup on the website for information on how to set up your environment.
Problems with current mobile app development methods
Let’s start with the problems developers and companies face with the current mobile app development methods.
A majority of mobile application based companies face the problem of maintaining separate teams for Android and iOS. This leads to code duplication, as well as a waste of resources and time for achieving the same goal. With the advent of Flutter, a single team can design applications for both Android and iOS, with unprecedented speed and performance.
The various designer-developer tangles are not new to those in the mobile app development industry. Different platforms have different UIs and it becomes a pain for the developer to not only concentrate on the differing UIs of the platforms but also code the main logic of the application. Simple UI changes like a different colour, font or style can hinder the actual app development as there is a long waiting time for rendering.
Solutions offered by Flutter
Now let’s focus on the solutions offered by the amazing Flutter team to these problems.
Flutter offers the same team the capability to design apps for both Android and iOS, with higher speed and better performance, reducing the need for companies to hire extra manpower. Higher synchronisation can be expected with a single team concentrated on a common goal. Also, an incredible uniformity can be achieved by the companies when developing their apps if a single team has worked on multiple platforms.
Thus, Flutter can rightfully boast of requiring almost half the development time and half the manpower to achieve twice the efficiency in developing mobile apps.
The Hot Reload feature in Flutter sorts out various designer-developer tangles. By running changes in milliseconds, the UI can be designed in an incredibly fast way, saving the time of the designers and the developers. Changing the colour of a page or an icon can seem too trivial for developers, yet is of utmost important for designers. With Flutter, both teams’ goals are achieved by Hot Reload, which basically delivers an updated design in record time.
The only disadvantage of Flutter is that you can now forget about the long coffee break you got while the app was kept to run!
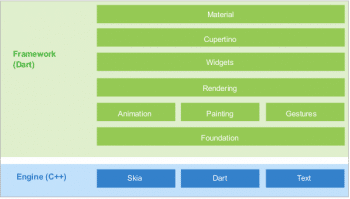
Some big players that use Flutter in production
Google has been using Flutter internally for many of its projects, way before it was opened to the public. Flutter has been used to build Google’s mobile sales tool app and also the Store Manager app for Google Shopping Express.
Outside Google, the teams that have started using Flutter are Hamilton Musical, Alibaba, AppTree, Abbey Road, etc. You can find a list of such apps at https://flutter.io/showcase/.
‘Hello World’ in Flutter
The easiest way to get introduced to any language is to understand the ‘Hello World’ code. So let’s get started.
import 'package:flutter/material.dart'; void main() => runApp(new MaterialApp( title: 'First Flutter App', home: MyApp(), )); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Demo App'), ), body: Center( child: Text('Hello World!'), ), ); } }
Importing the material.dart package: This is a pre-defined file to give the look and feel of material design to the app. We can materialise the app by using the widgets from the package (e.g., AppBar, Scaffold, etc).
MaterialApp: This consists of ‘home’, ‘route’, etc, as its parameters.
StatelessWidget: These widgets store the values of their parent widgets and use these values when the application is built.
Scaffold: This is a widget under the material app. It acts as a layout for the major material components.
MaterialApp can be built and run on an emulator or on a real device, similar to Android or iOS applications.
Yes! We have completed our first Flutter app and it’ll look exactly as shown in Figure 2.
Syntax and components
As we know, Flutter uses the Dart language for development. So let’s look at the basic syntax and usability of the various components of a Flutter app. Since we can’t cover all of it, I recommend that you go to the Flutter website and read the documentation, which is pretty good and also try out the Flutter Codelabs. They are very interesting and will help you learn faster.
Widgets
In Flutter, everything is a widget! As a result, it gets a lot easier for developers to visualise their applications in widgets.
The most commonly used widgets are listed below.
- Text: It lets you create styled text in your application:
Text( ‘Hello World!’, textAlign: TextAlign.center, style: new TextStyle(fontStyle: FontStyle.italic), )
- Row, Column: This let’s you create horizontal and vertical flexible layouts:
Row( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Icon(Icons.cake), Text(‘Happy Birthday!’) ], ) Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Icon(Icons.cake), Text(‘Happy Birthday!’) ], )
Stack: As opposed to Row and Column, Stack allows you to place widgets on top of one another.
Container: With its properties of padding, margins and various constraints, it lets you create a rectangular visual element for your application.
You can read about the basic widgets like Image, Icon, RiasedButton, etc, at https://flutter.io/widgets/basics/.
Stateful and stateless widgets: Widgets are divided into two types — stateful and stateless. You can select either depending upon the need of the application. So how do they differ?
To state it simply, stateless widgets are those that do not change with the changing state. But what is state? It is basically the information to be displayed. If you want to make changes to the content displayed by a stateless widget, you need to create a brand new widget.
Stateless widgets always build the same way, given a particular configuration.
Here’s an example that uses stateless widgets:
class Demo extends StatelessWidget { const Demo({ Key key }) : super(key: key); @override Widget build(BuildContext context) { return new Column(); } }
On the other hand, stateful widgets have mutable states. So when does one use them? They can be used when the UI is prone to dynamic changes.
Here’s an example that uses stateful widgets:
class Demo extends StatefulWidget { const Demo({ Key key }) : super(key: key); @override _DemoState createState() => new _DemoState(); } class _DemoState extends State<Demo> { @override Widget build(BuildContext context) { return new Column(); } }
Another type of widget is used when we have widgets subscribed to a single widget, and we want to efficiently pass on information to all the subscribed widgets when the single widget changes its state. That is, when an inherited widget changes, all the subscribed widgets will be re-drawn.
Learn more about inherited widgets at https://docs.flutter.io/flutter/widgets/InheritedWidget-class.html.
Gestures
Gestures can be in the form of a tap, a double tap, a long press, vertical and horizontal drags, etc.
Flutter provides us with the GestureDetector widget. For example:
new GestureDetector( onTap: () { setState(() { _birthday = true}); }, child: new Container( child: new Text(‘Happy Birthday!’), ), )
Flutter has plenty of features of its own, and can also be used for developing many interesting things such as animations and introducing internationalisation. Head over to flutter.io to develop your first Flutter app!
The best thing about Flutter is that developers from any platform can relate to Flutter right away! Many of Flutter’s components can be related to the components used presently in the Web, Android as well as iOS. As a result, it becomes easy for developers to relate their knowledge to Flutter.
Flutter for Web developers
Here is an example of HTML code used in Flutter for the Web:
.text{ font: 200 14px Arial; }
An example of Dart code is given below:
Text( “Happy Birthday”, style: new TextStyle( fontSize: 14.0 fontWeight: FontWeight.w200, fontFamily: “Arial” ), )
Another example of HTML code is:
.contain{ background-color: #26c6da; }
Another example of Dart code is:
Container( color: Colors.cyan[400] )
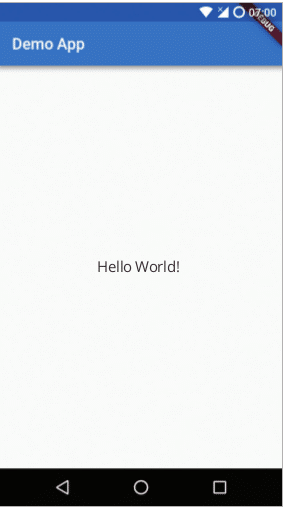
Flutter for Android developers
As an Android developer, you can easily apply your knowledge to build mobile apps with Flutter. It provides many components that are equivalent to the components in Android. So it becomes a lot easier to visualise your app in Flutter.
- Android Views are roughly equivalent to widgets in Flutter: View is the foundation of everything in Android and in Flutter, everything is a widget. But widgets are not strictly Views, as they are descriptions of the UI that gets converted into the actual View objects.
- Material library: Flutter uses widgets that implement the material design guidelines which are also used in Android.
- Draw/Paint: In Android, we use Canvas and Drawable. Flutter uses a similar Canvas API. So, for Android developers, these are familiar tasks in Flutter.
- Navigation: Android uses Intents to navigate between Activities and Flutter uses Navigator and Routes to navigate between screens.
Head over to the official website of Flutter at https://flutter.io/flutter-for-android/ to find out the various other similarities and differences with Android.
Flutter for iOS developers
As an iOS developer, you can easily apply your knowledge to build mobile apps with Flutter, which provides many components equivalent to those in the iOS framework. So it becomes a lot easier to visualise your app in Flutter.
- iOS UIView is roughly equivalent to widgets in Flutter: UIView is the foundation of everything in iOS and in Flutter, everything is a widget. But widgets are not strictly UIViews, as they are a description of the UI that gets converted into the actual UIView objects.
- Material library: Flutter uses widgets that implement the material design guidelines, which are optimised for iOS too.
- Opacity: Everything in iOS has .opacity or .alpha but in Flutter, we need to wrap the widgets in an Opacity widget.
- Navigation: In iOS, UINavigationController is used to manage the view controllers and Flutter uses Navigator and Routes to navigate between screens.
Head over to the official website of Flutter at https://flutter.io/flutter-for-ios/ to find out the various other similarities and differences with iOS.
Flutter for React Native developers
Here is an example of the JS code, where function can be the start point:
function demoCode() { //start }
In the Flutter code, the start point is always the ‘main’ function:
main(){ //start }
Here is another example of the JS code:
var nullVar = null; if (!nullVar ) { //do something }
In Flutter, the code is as follows:
var nullVar = null; if (nullVar == null) { //do something }
Head over to the official website of Flutter at https://flutter.io/flutter-for-react-native/ to find out the various other similarities and differences with React Native.
There’s a lot more to Flutter than what has been covered in this article. The native performance, amazing responsiveness, flexible UI and faster development makes it a perfect framework for mobile application development. Flutter has a pretty huge community and, with the latest release, it can be safely said that it is here to stay. The awesomeness of Flutter can only be known if you give it a try. The Google Codelabs (https://flutter.io/codelabs/) is a great starting point and will guide you thoroughly through the process.
This post clears my lots of confusion about app development. Thank you so much for this informative tricky post.