A pointer is a special kind of variable that holds the address of another variable. The same concept applies to function pointers, except that instead of pointing to variables, they point to functions. If you declare an array, say, int a[10];
then the array name a
will in most contexts (in an expression or passed as a function parameter) “decay” to a non-modifiable pointer to its first element (even though pointers and arrays are not equivalent while declaring/defining them, or when used as operands of the sizeof
operator). In the same way, for int func();
, func
decays to a non-modifiable pointer to a function. You can think of func
as a const
pointer for the time being.
But can we declare a non-constant pointer to a function? Yes, we can — just like we declare a non-constant pointer to a variable:
int (*ptrFunc) ();
Here, ptrFunc
is a pointer to a function that takes no arguments and returns an integer. DO NOT forget to put in the parenthesis, otherwise the compiler will assume that ptrFunc
is a normal function name, which takes nothing and returns a pointer to an integer.
Let’s try some code. Check out the following simple program:
#include<stdio.h> /* function prototype */ int func(int, int); int main(void) { int result; /* calling a function named func */ result = func(10,20); printf("result = %d\n",result); return 0; } /* func definition goes here */ int func(int x, int y) { return x+y; }
As expected, when we compile it with gcc -g -o example1 example1.c
and invoke it with ./example1
, the output is as follows:
result = 30
The above program calls func()
the simple way. Let’s modify the program to call using a pointer to a function. Here’s the changed main()
function:
#include<stdio.h> int func(int, int); int main(void) { int result1,result2; /* declaring a pointer to a function which takes two int arguments and returns an integer as result */ int (*ptrFunc)(int,int); /* assigning ptrFunc to func's address */ ptrFunc=func; /* calling func() through explicit dereference */ result1 = (*ptrFunc)(10,20); /* calling func() through implicit dereference */ result2 = ptrFunc(10,20); printf("result1 = %d result2 = %d\n",result1,result2); return 0; } int func(int x, int y) { return x+y; }
The output has no surprises:
result1 = 30 result2 = 30
A simple callback function
At this stage, we have enough knowledge to deal with function callbacks. According to Wikipedia, “In computer programming, a callback is a reference to executable code, or a piece of executable code, that is passed as an argument to other code. This allows a lower-level software layer to call a subroutine (or function) defined in a higher-level layer.”
Let’s try one simple program to demonstrate this. The complete program has three files: callback.c
, reg_callback.h
and reg_callback.c
.
/* callback.c */ #include<stdio.h> #include"reg_callback.h" /* callback function definition goes here */ void my_callback(void) { printf("inside my_callback\n"); } int main(void) { /* initialize function pointer to my_callback */ callback ptr_my_callback=my_callback; printf("This is a program demonstrating function callback\n"); /* register our callback function */ register_callback(ptr_my_callback); printf("back inside main program\n"); return 0; }
/* reg_callback.h */ typedef void (*callback)(void); void register_callback(callback ptr_reg_callback);
/* reg_callback.c */ #include<stdio.h> #include"reg_callback.h" /* registration goes here */ void register_callback(callback ptr_reg_callback) { printf("inside register_callback\n"); /* calling our callback function my_callback */ (*ptr_reg_callback)(); }
Compile, link and run the program with gcc -Wall -o callback callback.c reg_callback.c
and ./callback
:
This is a program demonstrating function callback inside register_callback inside my_callback back inside main program
The code needs a little explanation. Assume that we have to call a callback function that does some useful work (error handling, last-minute clean-up before exiting, etc.), after an event occurs in another part of the program. The first step is to register the callback function, which is just passing a function pointer as an argument to some other function (e.g., register_callback
) where the callback function needs to be called.
We could have written the above code in a single file, but have put the definition of the callback function in a separate file to simulate real-life cases, where the callback function is in the top layer and the function that will invoke it is in a different file layer. So the program flow is like what can be seen in Figure 1.
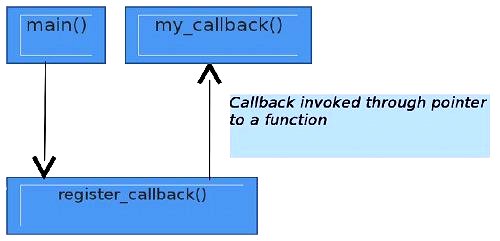
The higher layer function calls a lower layer function as a normal call and the callback mechanism allows the lower layer function to call the higher layer function through a pointer to a callback function.
This is exactly what the Wikipedia definition states.
Use of callback functions
One use of callback mechanisms can be seen here:
/ * This code catches the alarm signal generated from the kernel Asynchronously */ #include <stdio.h> #include <signal.h> #include <unistd.h> struct sigaction act; /* signal handler definition goes here */ void sig_handler(int signo, siginfo_t *si, void *ucontext) { printf("Got alarm signal %d\n",signo); /* do the required stuff here */ } int main(void) { act.sa_sigaction = sig_handler; act.sa_flags = SA_SIGINFO; /* register signal handler */ sigaction(SIGALRM, &act, NULL); /* set the alarm for 10 sec */ alarm(10); /* wait for any signal from kernel */ pause(); /* after signal handler execution */ printf("back to main\n"); return 0; }
Signals are types of interrupts that are generated from the kernel, and are very useful for handling asynchronous events. A signal-handling function is registered with the kernel, and can be invoked asynchronously from the rest of the program when the signal is delivered to the user process. Figure 2 represents this flow.
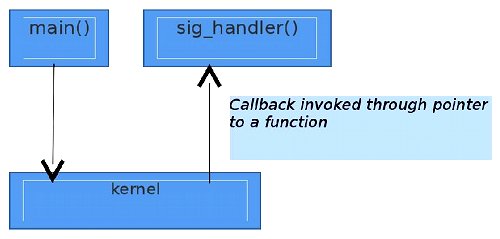
Callback functions can also be used to create a library that will be called from an upper-layer program, and in turn, the library will call user-defined code on the occurrence of some event. The following source code (insertion_main.c
, insertion_sort.c
and insertion_sort.h
), shows this mechanism used to implement a trivial insertion sort library. The flexibility lets users call any comparison function they want.
/* insertion_sort.h */ typedef int (*callback)(int, int); void insertion_sort(int *array, int n, callback comparison);
/* insertion_main.c */ #include<stdio.h> #include<stdlib.h> #include"insertion_sort.h" int ascending(int a, int b) { return a > b; } int descending(int a, int b) { return a < b; } int even_first(int a, int b) { /* code goes here */ } int odd_first(int a, int b) { /* code goes here */ } int main(void) { int i; int choice; int array[10] = {22,66,55,11,99,33,44,77,88,0}; printf("ascending 1: descending 2: even_first 3: odd_first 4: quit 5\n"); printf("enter your choice = "); scanf("%d",&choice); switch(choice) { case 1: insertion_sort(array,10, ascending); break; case 2: insertion_sort(array,10, descending); case 3: insertion_sort(array,10, even_first); break; case 4: insertion_sort(array,10, odd_first); break; case 5: exit(0); default: printf("no such option\n"); } printf("after insertion_sort\n"); for(i=0;i<10;i++) printf("%d\t", array[i]); printf("\n"); return 0; }
/* insertion_sort.c */ #include"insertion_sort.h" void insertion_sort(int *array, int n, callback comparison) { int i, j, key; for(j=1; j<=n-1;j++) { key=array[j]; i=j-1; while(i >=0 && comparison(array[i], key)) { array[i+1]=array[i]; i=i-1; } array[i+1]=key; } }
Wrapping it up
The tutorial describes how callbacks are implemented in C. In C++ the same goal can be achieved through template functors. Although callbacks are very hard to trace in large real-world programs, life should be a little easier after going through this introductory article. Just remember, callback is nothing but passing the function pointer to the code from where you want your handler/callback function to be invoked. Happy hacking!
Reference
- Introduction to Algorithms, 3/e by Thomas H Cormen, Charles E Leiserson, Ronald L Rivest and Clifford Stein
- Linux System Programming by Robert Love
- Function Pointers in C++
If you don’t give example of QuickSort then youR whole point of calling yourself Linux for you is stupid
case 2,forget break;
ya stupid only nothing useful total confusing
what is difference b/n function returning int* vs pointer to function returning int
int *func1(float);
int *func1(float);
consider a function:
main(){
int &ptr;
ptr=func(); //assigns the address of a to ptr;
printf(“%d” ,*ptr); will print the value of a;
}
int *func(){
int a=10;
return &a; // we are returning the address of a(which is an integer)
}
The above function returns a pointer to a variable which is an integer.This function thus called as “function returning int*)..
“Pointer to function returning int” means a function which returns an integer.
difference b/n
int *func1(float);
int (*func2)(void);
int *func1(float)
– a function func1 which takes an argument in float and returns a pointer to an integer variable or address of that variable;
int (*func2)(void)
– a function func2 is a function pointer which takes no argument and returns an integer.
great explanation. i had been struggling reading a piece of code I had with callbacks in C. This tutorial cleaned my thought and made life much easier. Thanks!
superb!!thank you
nice artical…very helpful
thanks, at last I understand callbacks :)
Thanks a lot for the article!
This is really good.
Great article with perfect examples!
Great Article, nicely explained
Thanks sir
In the first example, what would be the benefit of using a callback, why can’t my_callback(void) be just called in the main method? Why a pointer to a function is benefitial in some cases? Thanks for the article!
1) You can pass function as an argument (code reduced, complexity reduced)
2) Can be called at run time instead of compile time. ( some event or interrupt based)
Yep.
please give one more example for calling a callback function asynchronously
Very Nice Explainition :)
None of the code explains why or how exactly callback functions are being used. Would be better if you explain with a program that handles asynchronous events with callback functions.
Hi, Dibyendu Roy,
Your article clear my concept on “Function Pointer”, I really like your article, Thanks for sharing this useful information.
Nicely written,
Thanks for sharing !!