This article will delight newbies who want to get a foothold in the programming world. It introduces readers to the fascinating world of Python, which is a very versatile programming language.
Computers, today, come with immense disk space, multi-core processors and high speed Internet connectivity. And they use computer languages that interact with the operating system and execute processes at a high speed. One such language is Python, which is used as a high level programming language. It was first released in 1991 by Guido van Rossum, an ex-employee of Google. It is quite easy to learn, as it uses English keywords frequently, unlike the heavy syntaxes of other languages. Moreover, programmers can code the logic using fewer lines of code than what is required in other programming languages like C or Java. The unique design, notably, using white space indentation to delimit code blocks, makes Python more readable and clean.
Python is an interpreted, interactive and object-oriented scripting language. The Python code is processed by the processor at run time, i.e., it does not need to be compiled as in Java, which is compiled to get the .class file. The term interactive implies that you can use the Python prompt to write the code, which interacts with the interpreter, and by encapsulating the code within objects, it follows the OOPS principle. The other features that make Python cool are that it is extendable, i.e., it can run on a wide range of hardware with the same user interface; it is scalable, supporting very large programs; and it has user interfaces for UNIX, Windows and Mac. It also has efficient high-level data structures and extensive standards-free libraries, which make programming easy; hence, it is considered the language for beginners.
In this article, we will learn how to install, set up and use Python. The cool thing about learning Python is that there are no prerequisites. Even if you are a novice to programming you can become a pro coder within a month. To start with, download and install Python.
For this article, we are using Python 2.7. The above link also contains Python for Windows and Mac. On most Linux boxes, Python is pre-installed. For Windows, download Python and run the .msi file. Upon completion, add the environment variable named ‘PYTHON’ and its value as the directory where Python is (in this case,’C:\Python27′), as shown in Figure 1. We can verify whether Python has been installed correctly by running the python – version command in the command line. This will give you the current version of Python running on your computer, as shown in Figure 2. To create Python files, use any text editor of your choice. For this article, we will be using the Sublime text editor.
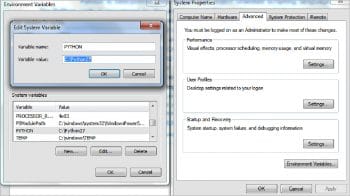
The cliché of programming languages is ‘Hello World’; so let’s get started by printing it in the console. Open the command prompt, type Python and execute it. Now run the command:
print (“Hello world”).
This will print ‘Hello World’ in the console as shown in Figure 3. Python, being a scripting language, can also be executed by writing a group of individual statements, which make a single code block called a ‘suite’ in a file, and can be saved with the .py extension.
Now let’s learn about the building blocks of Python, like the identifiers, keywords, user input processes, comments, etc. Identifiers are the names given to classes, variables, functions, etc. These are case sensitive and start with the letters A-Z, a-z or an underscore. There are some naming conventions in Python, which are listed below:
- An identifier starting with an underscore means that it’s private, and a double underscore means it is strongly private.
- The class name must start with upper case. Python has some reserved words called keywords which have special meanings. Examples of keywords are and, finally, Class, def, if, else, elif, etc. Unlike Java or C, which use braces to wrap lines of code, Python uses indentation to denote the block of code that is tightly mapped. In Python, statements usually end with a new line, but if the statement gets carried to the new line, then one uses the ‘\’ backslash to show the continuation of the line. A single line comment is denoted by ‘#’ and a multi-line comment can be wrapped in triple quotes.
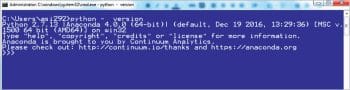
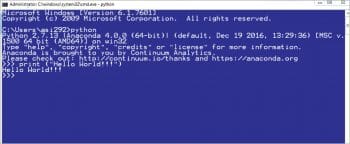
The data types used in Python are numbers, strings, lists, tuples and the dictionary. The number data type stores the numeric value and is immutable in nature, i.e., upon changing the value, a new object is created and the older one is kept for automatic garbage collection. For example, variable = 10. The single quote or double quotes are used to denote the string. For example, varA = ‘Hello World’, varB = “Python is cool”. Python does not support character types, but treats the characters as strings of length one. Coming to the list, it consists of comma-separated values which could be of any type and it is represented as [,,,,]. All values are enclosed between ‘[‘ and ‘]’. A list object is a mutable data type, which means it can’t be hashed, and modifying an existing list will not result in a new list object—the memory address will not be changed either. For example: def_list = [1, 2,”1″,”100″,”Python”,”Anne”].
The next is a tuple, which has comma-separated values that could be of any type, enclosed between ‘(‘ and ‘)’. This, too, is an immutable data type and can be used as the key in a dictionary. For example: def_tuple = (1,2,”1″,”100″,”Python”,”Anne”). The dictionary in Python contains the key value pair in which the key is immutable, and the value can be anything; the key value is separated by a colon ‘:’. Dictionaries work on the hashing principle. For example: dictB = dict(). Let us now create a new file in the Sublime text editor with the following commands:
# An Empty Dict dictA = dict() # same as dictA = {} dictB = dict() # same as dictB = {} # Adding values to it for i in [1,3,2,4,8,9,5,6,7]: dictB[i] = i # Adding values to a dict # Let us use a simple for loop to add values to a dict for i in xrange(10): dictA[i] = i + 10 print dictA,'\n\n',dictB,'\n\n' ''' Define a simple list with multiple datatypes ''' def_list = [1,2,"1","100","Python","Anne","A!@345<>_()",True,False,{1:100,2:200,3:300},range(10)] #Now create a variable vara = def_list #Modification of vara will result in modifying def_list vara.append("Hero") print "Address of vara and def_list %s and %s "%(id(vara),id(def_list)),'\n\n' print "vara = %s "%(vara),'\n\n' print “def_list = %s “%(def_list),’\n\n’ # Define a simple tuple with multiple datatypes def_tuple = 1,2,”1”,”100”,”Python”,”Anne”,”A!@345<>_()”,True,False,{1:100,2:200,3:300},range(10) # Print and view the tuple print “Printing the tuple - “ , def_tuple, ‘\n\n’ print “Printing the tuple’s address - “ , id(def_tuple),’\n\n’# The address is subject to change
Once this is done, save the file with the .py extension. In this case, it is saved as PythonPractice.py. In the command prompt, browse to the location where the file is saved and execute the command Python PythonPractice.py (shown in Figure 4); this displays the result on the console (as shown in Figure 5).
Python is famous for its functional programming. A function is a block of organised, reusable code that is used to perform a task. It provides better modularity because of the ability to be reused.
There are a few rules for writing the function, which are:
a) It begins with the ‘def’ keyword, followed by the name and parentheses, which can be used to pass the input parameters.
b) A code block within every function starts with a colon (:) and is indented. A simple example of a function is shown below:
#A Function to print the sum of numbers def funcA(a,b,c): #Add a,b,c and return the result sum_numbers = a + b + c return sum_numbers
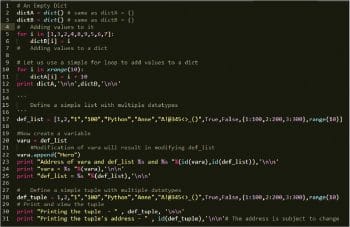
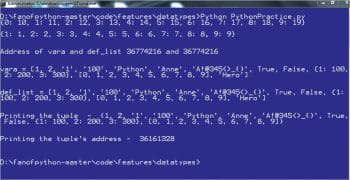
This can be called by executing print funcA(100,200,300), which prints the result 600 in the console.
There are many other useful features of Python like modules, inheritance, multi-processors, exceptions, file input and output, all of which make Python a high level and easy programming language. Python also has classes and objects, multi-threading, database access, networking, emails and many more features, which can be learned while exploring the advanced features.
[…] This article introduces readers to the fascinating world of Python, which is a very versatile programming language. Read more […]
Python is very useful and interesting but python is difficult.