Code it, commit it, forget it! GitHub Actions and Docker Hub ensure your deployment hits production faster than you can say ‘push’.
In software development, speed and efficiency are everything. Continuous deployment, a practice that automatically deploys new code changes to production, has become a cornerstone of modern development methodologies. To streamline this process, developers often turn to powerful tools like Docker Hub and GitHub Actions.
Let’s explore how Docker Hub and GitHub Actions work together to streamline the continuous deployment workflow.
What is Docker Hub?
Docker Hub is a cloud-based repository that serves as a central location for storing, managing, and distributing Docker images. Think of it as a library for pre-packaged applications where you can find images created by various individuals and organisations.
Docker images play a crucial role in continuous deployment (CD) workflows. They provide a consistent and isolated environment for your application, ensuring that it runs the same way regardless of the underlying infrastructure. This standardisation simplifies the deployment process and reduces the risk of errors.
What is GitHub Actions?
GitHub Actions is a CI/CD automation tool built into GitHub. It allows you to automate various tasks within your software development workflow, such as building, testing, and deploying your code. You can create custom workflows using simple declarative syntax, and GitHub provides a wide range of prebuilt actions to streamline your processes.
GitHub Actions offers a powerful way to automate your software development pipeline. Table 1 lists the key roles it plays.
Role of GitHub Actions |
What it automates |
|
Automates testing and code integration to ensure quality before merging. |
|
Streamlines and automates application deployments across various environments. |
|
Executes unit, integration, and end-to-end tests on every code update. |
|
Automatically checks and enforces consistent code style and formatting. |
|
Handles automatic updates and version control of project dependencies. |
|
Detects and flags vulnerabilities in code automatically. |
|
Automates tasks like labelling and commenting on issues and pull requests. |
|
Builds and deploys Docker containers in an automated manner. |
|
Enables the automation of any repetitive or manual task with custom workflows. |
Table 1: Key roles of GitHub Actions
How Docker Hub fits into continuous deployment
To set up automated image builds using Docker Hub and facilitate continuous deployment, you can link your GitHub repository with Docker Hub. Here’s a simple example of how you can do this.
Create a new repository
- Log into your Docker Hub account and create a new repository by clicking the ‘Create Repository’ button.
- Provide a name for your repository (e.g., myapp) and select whether it should be public or private.
Link your GitHub repository
- In the repository settings, locate the ‘Builds’ section and click on ‘Configure Automated Builds’.
- You will be prompted to connect your GitHub account. Authorise Docker Hub to access your GitHub repositories.
- Select the repository you want to link (e.g., username/myapp).
Specify the branch
- In the automated builds configuration, you can specify which branch to monitor (e.g., main or develop).
- When code is pushed to this branch, Docker Hub will automatically build a new image.
- Once set up, every push to the linked branch will trigger Docker Hub to build and tag a new image automatically.
- For example, if a developer pushes changes to the main branch, Docker Hub will execute the build process based on the Dockerfile in the repository.
Here’s an example of a simple Dockerfile for a Node.js server:
# Dockerfile FROM node:14 # Set the working directory WORKDIR /app # Copy package.json and install dependencies COPY package.json ./ RUN npm install # Copy the rest of the application code COPY . . # Expose the port EXPOSE 3000 # Start the application CMD [“npm”, “start”]
To deploy the new Docker image automatically upon receiving a webhook from Docker Hub, you can set up a simple Node.js server to listen for the webhook:
// server.js const express = require(‘express’); const { exec } = require(‘child_process’); const app = express(); const PORT = 3000; // Middleware to parse incoming JSON requests app.use(express.json()); // Endpoint to handle Docker Hub webhook app.post(‘/webhook’, (req, res) => { console.log(‘Received webhook:’, req.body); // Extract repository name from webhook payload const repository = req.body.repository.repo_name; // Pull the new image from Docker Hub exec(`docker pull myusername/${repository}:latest`, (err, stdout, stderr) => { if (err) { console.error(`Error pulling image: ${stderr}`); return res.status(500).send(‘Failed to pull new image’); } // Restart the Docker container (assuming it’s named ‘myapp’) exec(‘docker stop myapp && docker rm myapp’, (err) => { if (err) { console.error(`Error stopping container: ${stderr}`); return res.status(500).send(‘Failed to stop old container’); } exec(`docker run -d --name myapp -p 3000:3000 myusername/${repository}:latest`, (err) => { if (err) { console.error(`Error starting new container: ${stderr}`); return res.status(500).send(‘Failed to start new container’); } console.log(‘Successfully deployed the new image’); res.status(200).send(‘Deployment successful’); }); }); }); }); // Start the server app.listen(PORT, () => { console.log(`Server listening on port ${PORT}`); });
By linking your GitHub repository to Docker Hub and configuring automated builds, you enable a smooth continuous deployment process. Every time code changes are pushed to the specified branch, Docker Hub will automatically build a new image. The provided Node.js server listens for webhooks, and can pull the new image and redeploy the application seamlessly.
GitHub Actions for continuous integration and deployment
Creating a CI/CD pipeline with GitHub Actions involves defining workflows in YAML files that specify the steps to be executed in response to certain events. The workflows are stored in the .github/workflows/ directory of your repository.
- Define a workflow: You create a YAML file to define the workflow, including its name, trigger events (like push or pull_request), and jobs.
- Specify jobs and steps: Each job can run in parallel and consists of multiple steps, which can include actions, shell commands, or scripts.
Table 2: Key features of GitHub Actions
Feature | Functionality |
Event driven | Triggers workflows based on specific events, such as commits, pull requests, or releases. |
Matrix builds | Executes tests or builds across multiple environments or versions of dependencies simultaneously. |
Reusable actions | Leverages prebuilt actions from the community to simplify and streamline workflows. |
Integration with Docker | Seamlessly builds, tests, and pushes Docker images within GitHub Actions. |
Here’s an example of a GitHub Actions workflow that automates the process of building a Docker image, running tests, and pushing the image to Docker Hub.
Create a Dockerfile: First, ensure you have a Dockerfile for your application. Here’s an example for a simple Node.js application:
# Dockerfile FROM node:14 WORKDIR /app COPY package.json ./ RUN npm install COPY . . EXPOSE 3000 CMD [“npm”, “start”]
Create the GitHub Actions workflow: In your repository, create a new file .github/workflows/docker-ci-cd.yml and define the workflow as follows:
# .github/workflows/docker-ci-cd.yml name: CI/CD Pipeline for Docker on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Set up Docker Buildx uses: docker/setup-buildx-action@v1 - name: Log in to Docker Hub uses: docker/login-action@v2 with: username: ${{ secrets.DOCKER_HUB_USERNAME }} password: ${{ secrets.DOCKER_HUB_PASSWORD }} - name: Build Docker Image run: | docker build -t myusername/myapp:latest . - name: Run Tests run: | docker run --rm myusername/myapp:latest npm test - name: Push Docker Image run: | docker push myusername/myapp:latest
Let’s explain this. The workflow is triggered when there are pushes to the main branch. In response, the build job runs on the latest version of Ubuntu.
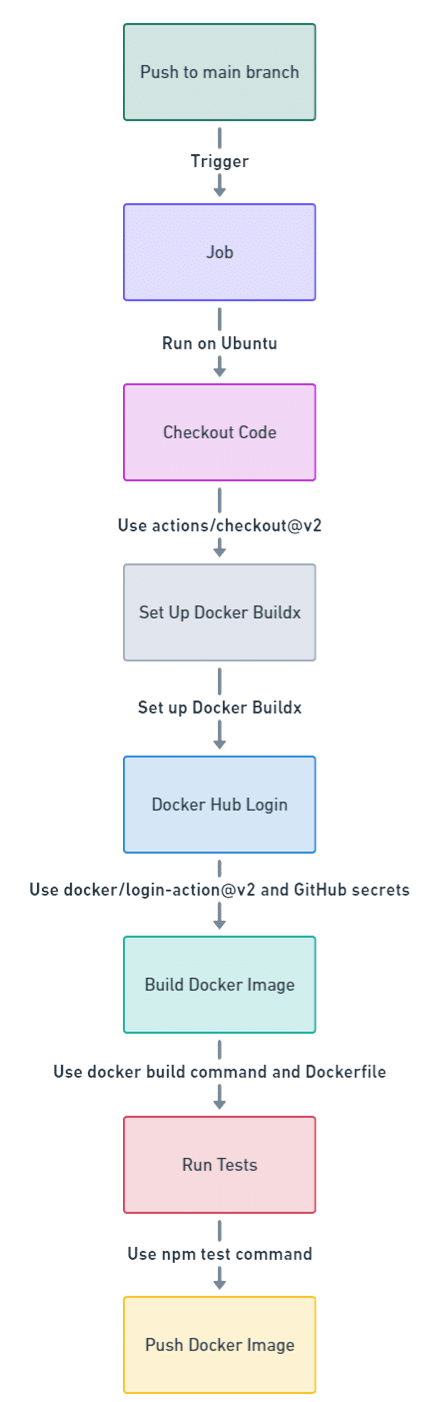
First, the actions/checkout@v2 step checks out the repository code to ensure that the workflow has access to the latest changes.
Next, this action sets up Docker Buildx, which allows for advanced build capabilities, enabling the use of features like multi-platform builds.
Following that, the docker/login-action@v2 step logs into Docker Hub using credentials stored as secrets in GitHub. It’s important to store your Docker Hub credentials in the repository settings under Secrets for security.
After logging in, the workflow runs the docker build command to create the Docker image based on the provided Dockerfile.
Subsequently, the workflow runs tests within a new container using the npm test command. This assumes you have defined tests in your package.json.
Finally, the newly built image is pushed to Docker Hub using the docker push command, making it available for deployment.
Benefit | Overview |
Simplified automation | – Streamlined deployment processes
– Reduced manual intervention – Faster feedback loops |
Scalability and flexibility | – Customisable workflows for different environments
– Scalable architecture for increased demand – Seamless multi-environment management |
Increased reliability | – Consistent testing and deployment
– Early error detection through automated testing – Improved rollback capabilities for quick recovery |
Table 3: The benefits of combining Docker Hub and GitHub Actions
Best practices for Docker Hub and GitHub Actions in CD
When integrating Docker Hub and GitHub Actions into your continuous deployment (CD) workflows, adhering to best practices is crucial for ensuring security, efficiency, and reliability. Here are some essential best practices to consider when using these tools in your CD processes.
Securing Docker images
- Regularly scan images for vulnerabilities using tools like Docker Scout or Snyk, and automate scanning in your CI/CD pipeline.
- Integrate security tests and linting checks in GitHub Actions to catch vulnerabilities early.
- Use GitHub Secrets to store sensitive information securely, avoiding hardcoding in your codebase.
Optimising Docker images
- Use lightweight base images and remove unnecessary files to reduce image size and speed up deployments.
- Separate build and runtime environments to create smaller final images, copying only necessary artifacts.
- Structure your Dockerfile to maximise cache efficiency, speeding up build times.
Monitoring and logging
- Use solutions like Prometheus and Grafana to track application performance and health.
- Implement logging services (e.g., ELK Stack) to aggregate logs for better visibility and troubleshooting.
- Analyse monitoring data to identify trends and improve CD workflows.
As we have seen, by integrating Docker Hub and GitHub Actions, teams can significantly reduce manual intervention, accelerate development workflows, and enable faster feedback and more reliable software releases. This collaboration is a game changer for organisations looking to improve their software delivery pipeline and stay ahead of the competition.
If you’re ready to take your continuous deployment process to the next level, I encourage you to explore Docker Hub and GitHub Actions. This tutorial has provided a foundation, but there’s more to discover. Start experimenting with these tools and unlock the full potential of your software development pipeline.
Happy coding!